Voiced by Amazon Polly |
Overview
Python decorators are a powerful feature that allows you to modify or extend the behavior of functions or classes without changing their source code. They are essentially functions that wrap around other functions or classes and can be used to add new functionality or modify the existing behavior of the wrapped object.
Decorators are typically defined using the ‘@’ symbol followed by the decorator’s name and placed directly above the function definition. When the function is called, the decorator function is applied before it is executed.
The decorator takes in a function, includes a few functionalities, and returns it. Decorators permit you to expand and alter the behavior of a callable (methods, classes, and functions) without permanently altering the callable itself. It is a callable that takes a callable as input and returns another callable.
It’s also known as metaprogramming since a portion of the program tries to alter another portion at compile time.
Any adequately generic functionality you can track onto an existing function’s behavior makes an incredible utilization case for decoration. It includes the following
- Implementing Access control and authentication
- Rate-limiting
- Logging
- Instrumentation and timing functions
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Introduction
- Functions in Python
One of the foremost effective highlights of Python is that everything is an object, including functions. It is an organized and reusable code to perform a single related activity. Functions provide superior modularity for your application. They can be assigned to variables and passed to and returned from the other functions.
- Functions can be defined inside the other functions
Child Functions can capture the parent function’s local state (lexical closures)
A Simple Python Program to Understand the Concept of Decorators
This function illustrates the division of two numbers with two parameters, a and b.
- def smart_divide:
There are a couple of things to note here. Firstly, a decorator is characterized as a function and behaves like a function. It’s best to think of it as a function, and more critically, the argument a decorator acknowledges is the function it is decorating. Also, the name of the decorator can be anything. A decorator can also acknowledge multiple arguments.
- def inner(a,b):
This is often likely the most confusing portion of the code. A decorator must always return a function with a few functionalities to the original function. This is often commonly referred to as the wrapper function. This new function will replace the first function, so it must acknowledge precisely the same number of arguments the original function has (in this case, two). So certainly, this decorator will not enhance a function that doesn’t have two parameters accurately, although there are ways to induce around this using *args.
If the denominator equals zero, we will receive the following output, and your code attempts to divide a given number by zero. ZeroDivisionError
Let’s make a decorator check for this case that will cause the error.
This new execution will return None if the error condition emerges.
Output:
Let’s try to understand it with another decorator that does something and alters the
the behavior of the decorated function.
Example 1.1: Basically, it converts the results of the decorated function to lowercase letters:
1 2 3 4 5 6 |
def lowercase(func): def wrapper(): original_string = func() converted_string = original_string.lower() return converted_string return wrapper |
Rather than simply returning the input as the null decorator did, this lowercase decorator characterizes a new function on the closure and uses it to wrap the input function to alter its behavior at call time. The wrapper closure has got to the undecorated input function and is free to execute extra code before and after calling it. Until now, the decorated function has never been executed, and you’ll want the decorator to be able to alter the behavior of its input function when it eventually gets called.
Time to explore the lowercase decorator,
1 2 3 4 5 |
@lowercase def greet(): return "HELLO WORLD" >> greet() ‘hello world’ |
Let’s take a closer picture at what just happened here. Our lowercase decorator returns a different function object when it decorates a function.
1 2 |
>>> greet <function __main__.lowercase.<locals>.wrapper() at 0x20e9f750> |
1 2 |
>>> lowercase(greet) <function __main__.lowercase.<locals>.wrapper() at 0x67ad02f82> |
As you saw earlier, it needs to do that to alter the decorated function’s behavior when it finally gets called. The lowercase decorator is a function itself. It’s the only way to impact the future behavior of an input function it decorates, i.e., replace/wrap the input function with a closure.
That’s why lowercase characterizes and returns the closure function (another function) that can be called later, running the initial input function and modifying its result. Decorators alter the behavior of a callable through a wrapper closure, so you do not have to alter the original permanently. The original callable isn’t permanently adjusted; its behavior changes only when decorated.
Conclusion
This feature lets the user tack on reusable building blocks like other instrumentation and logging into the existing functions and classes. It makes decorators such an effective tool in Python that it’s frequently used in the standard library and third-party packages.
Making IT Networks Enterprise-ready – Cloud Management Services
- Accelerated cloud migration
- End-to-end view of the cloud environment
About CloudThat
CloudThat is also the official AWS (Amazon Web Services) Advanced Consulting Partner and Training partner and Microsoft gold partner, helping people develop knowledge of the cloud and help their businesses aim for higher goals using best in industry cloud computing practices and expertise. We are on a mission to build a robust cloud computing ecosystem by disseminating knowledge on technological intricacies within the cloud space. Our blogs, webinars, case studies, and white papers enable all the stakeholders in the cloud computing sphere.
Drop a query if you have any questions regarding Python Decorators and I will get back to you quickly.
To get started, go through our Consultancy page and Managed Services Package that is CloudThat’s offerings.
FAQs
1. What are some use cases for Python Decorators?
ANS: – Adding functionality like caching to functions, timing and logging, and modifying the behavior of the classes, functions, and methods.
2. What is a chain decorator in Python?
ANS: – Several decorators can be used inside a function by “chaining” them together. We can add many decorators to a function in Python.
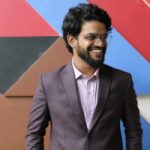
WRITTEN BY Anirudha Gudi
Anirudha Gudi works as Research Associate at CloudThat. He is an aspiring Python developer and Microsoft Technology Associate in Python. His work revolves around data engineering, analytics, and machine learning projects. He is passionate about providing analytical solutions for business problems and deriving insights to enhance productivity.
Comments