Overview
Python is a popular programming language for developing web applications, scientific computing, data analysis, and automation tasks. Python’s design philosophy emphasizes code readability and simplicity, making it an ideal language for beginners and experienced developers. One of the essential features of Python is its concurrency model, which allows you to execute multiple tasks simultaneously. In this blog post, we will explore two popular concurrency models in Python: Asyncio and Threading.
Concurrency
Concurrency is a programming concept that enables multiple tasks to execute simultaneously within a program.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Python's Concurrency Models: Asyncio and Threading
Python provides two popular concurrency models to achieve concurrency: Asyncio and Threading. Both models have their unique features, advantages, and disadvantages. Now, let’s examine these concepts more closely to understand how they work and how they can be applied in Python programming.
Asyncio
Asyncio is a Python library that provides an event-driven, non-blocking I/O model. Asyncio allows you to write highly concurrent and efficient code in a structured way. The key concept of Asyncio is coroutines, which are like generators but provide a more efficient way to write asynchronous code.
Asyncio’s core principle is the event loop, which continuously checks for events and executes the appropriate callback function. Asyncio provides various functions to interact with the event loop, such as asyncio.run() and asyncio.create_task(). These functions allow you to run and manage coroutines efficiently.
Asyncio is useful for I/O-bound tasks, such as network programming, where the program spends most of its time waiting for I/O operations to complete. Asyncio’s non-blocking I/O model enables the program to execute other tasks while waiting for I/O operations to complete, making it an efficient way to handle I/O-bound tasks.
Threading
Threading is a Python library that allows you to execute multiple threads within a single program. Threads are lightweight, independent units of execution that run concurrently with other threads in the same process. Each thread runs in its own memory space but shares the same system resources as other threads in the same process.
Threading is useful for CPU-bound tasks, such as scientific computing, where the program spends most of its time executing CPU-intensive operations. Threading allows you to utilize multiple CPUs and cores to perform tasks simultaneously, improving the program’s performance.
Threading provides various functions to create and manage threads, such as threading.Thread() and threading.Lock(). These functions allow you to create and manage threads efficiently.
Choosing Between Asyncio and Threading
Choosing between asyncio and threading in Python can depend on the specific needs of your application. Here are some points to consider when deciding between the two concurrency models:
When to use Asyncio:
- Your application relies heavily on I/O operations, such as network requests or files I/O.
- Your application needs to handle a large number of concurrent connections or requests.
- Your application needs to be scalable and handle a large amount of traffic.
- Your application can be broken down into small, independent tasks that can be executed sequentially.
When to use Threading:
- Your application relies heavily on CPU-bound operations, such as mathematical calculations or data processing.
- Your application must execute tasks in parallel, where each thread can execute its instructions independently.
- Your application must interact with external resources that do not support asynchronous I/O, such as database drivers or third-party libraries.
Conclusion
Python provides two popular concurrency models, Asyncio and Threading, to achieve concurrency. Both models have their unique features, advantages, and disadvantages. Choosing between Asyncio and Threading depends on the type of task you want to perform. Asyncio is useful for I/O-bound tasks, while Threading is useful for CPU-bound tasks. Understanding these concurrency models’ strengths and weaknesses can help you write more efficient and scalable Python code.
Empowering organizations to become ‘data driven’ enterprises with our Cloud experts.
- Reduced infrastructure costs
- Timely data-driven decisions
About CloudThat
CloudThat is an official AWS (Amazon Web Services) Advanced Consulting Partner and Training partner and Microsoft Gold Partner, helping people develop knowledge of the cloud and help their businesses aim for higher goals using best-in-industry cloud computing practices and expertise. We are on a mission to build a robust cloud computing ecosystem by disseminating knowledge on technological intricacies within the cloud space. Our blogs, webinars, case studies, and white papers enable all the stakeholders in the cloud computing sphere.
Drop a query if you have any questions regarding Python, I will get back to you quickly.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. Can you use both asyncio and threading in the same Python application?
ANS: – You can use asyncio and threading together in Python. But mixing concurrency models can lead to issues like blocking or unsafe access to shared resources. So, it’s important to be careful and coordinate properly.
2. How do you handle errors and exceptions when working with asyncio and threading in Python?
ANS: – Proper error and exception handling is crucial when using asyncio and threading in Python. In asyncio, try/except blocks can handle exceptions in coroutines, while threading requires synchronization primitives like locks to prevent race conditions and ensure thread-safe access to shared resources.
3. How can you measure your Python application's performance of asyncio and threading?
ANS: – You can measure the performance of asyncio and thread in your Python application using profiling tools such as cProfile or PyCharm’s built-in profiler. These tools can help you identify performance bottlenecks in your code and determine which concurrency model is better suited for your specific use case.
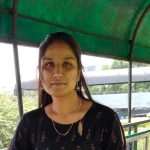
WRITTEN BY Anusha
Anusha works as Research Associate at CloudThat. She is an enthusiastic person about learning new technologies and her interest is inclined towards AWS and DataScience.
Comments