Overview
Optimizing game performance is a pivotal aspect of Unity development, especially considering the continuous creation and destruction of game objects that can lead to performance bottlenecks. Object Pooling, a design pattern managing a pool of reusable objects, emerges as a powerful solution. This blog post delves deeper into Object Pooling in Unity using C#, emphasizing its advantages and offering comprehensive insights into its implementation.
The Need for Object Pooling
Memory Efficiency
The conventional approach of instantiating and destroying objects during runtime contributes to memory fragmentation. Object Pooling resolves this issue by reusing existing objects, reducing the need for constant memory allocation and deallocation.
Performance Enhancement
The dynamic creation and destruction of objects incur performance costs. Object Pooling mitigates this overhead by recycling objects, resulting in a smoother gameplay experience and reduced CPU load.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Implementing Object Pooling in Unity
Pool Initialization
Creating a pool of objects during game initialization involves instantiating them and setting them as inactive. Let’s enhance the example by allowing customization of object properties during initialization:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
```csharp void InitializePool() { objectPool = new List<GameObject>(); for (int i = 0; i < poolSize; i++) { GameObject obj = Instantiate(prefab); obj.SetActive(false); // Additional customization obj.GetComponent<YourCustomComponent>().Initialize(); objectPool.Add(obj); } } ``` |
Object Activation
When a new object is required, retrieve one from the pool, activate it, and allow for further customization:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
```csharp public GameObject GetObjectFromPool(Vector3 position, Quaternion rotation) { foreach (GameObject obj in objectPool) { if (!obj.activeInHierarchy) { obj.SetActive(true); obj.transform.position = position; obj.transform.rotation = rotation; // Additional customization obj.GetComponent<YourCustomComponent>().Activate(); return obj; } } // If no inactive object is found, create a new one GameObject newObj = Instantiate(prefab, position, rotation); // Additional customization newObj.GetComponent<YourCustomComponent>().Initialize(); objectPool.Add(newObj); return newObj; } ``` |
Object Deactivation
Instead of destroying objects, deactivate them when they are no longer needed, allowing for proper cleanup:
1 2 3 4 5 6 7 |
```csharp public void ReturnObjectToPool(GameObject obj) { obj.GetComponent<YourCustomComponent>().Deactivate(); obj.SetActive(false); } ``` |
C# Code Example
Continuing from the previous example, let’s introduce an event system to notify objects when they are activated or deactivated:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
```csharp public class YourCustomComponent : MonoBehaviour { public delegate void ObjectEvent(GameObject obj); public static event ObjectEvent OnObjectActivated; public static event ObjectEvent OnObjectDeactivated; public void Initialize() { // Initialization logic } public void Activate() { // Activation logic OnObjectActivated?.Invoke(gameObject); } public void Deactivate() { // Deactivation logic OnObjectDeactivated?.Invoke(gameObject); } } ``` |
Best Practices
Prefab Variation
Consider managing pools for different prefab variations if your game involves a variety of objects with distinct behaviors.
Performance Profiling
Utilize Unity’s profiling tools to analyze and optimize the impact of object pooling on your game’s performance.
Example for using Unity's UI system
A simple example using Unity’s UI system
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 |
```csharp using UnityEngine; using UnityEngine.UI; using System.Collections.Generic; public class UIPoolManager : MonoBehaviour { public GameObject uiPrefab; public int poolSize = 10; private List<GameObject> uiObjectPool; void Start() { InitializeUIPool(); } void InitializeUIPool() { uiObjectPool = new List<GameObject>(); for (int i = 0; i < poolSize; i++) { GameObject uiElement = Instantiate(uiPrefab, transform); uiElement.SetActive(false); uiObjectPool.Add(uiElement); } } public GameObject GetUIElementFromPool(Vector2 position) { foreach (GameObject uiElement in uiObjectPool) { if (!uiElement.activeInHierarchy) { uiElement.SetActive(true); uiElement.GetComponent<RectTransform>().anchoredPosition = position; return uiElement; } } // If no inactive UI element is found, create a new one GameObject newUIElement = Instantiate(uiPrefab, transform); newUIElement.GetComponent<RectTransform>().anchoredPosition = position; uiObjectPool.Add(newUIElement); return newUIElement; } public void ReturnUIElementToPool(GameObject uiElement) { uiElement.SetActive(false); } } ``` |
In this example, the UIPoolManager
handles the pooling of UI elements, and the GetUIElementFromPool
method is responsible for activating and positioning UI elements on demand. The ReturnUIElementToPool
method deactivates UI elements when they are no longer needed, making them available for reuse. Adjust the code according to your specific UI element requirements.
To further enrich your understanding, explore scenarios like pooling for UI elements or integrating Object Pooling with Unity’s Job System for parallelized performance gains. Experiment with asynchronous loading to pre-warm object pools, ensuring a seamless experience from the game’s outset.
Conclusion
With a proactive approach to customization and a keen eye for performance profiling, you can achieve a finely tuned, optimized, and enjoyable player experience.
Drop a query if you have any questions regarding Unity and we will get back to you quickly.
Making IT Networks Enterprise-ready – Cloud Management Services
- Accelerated cloud migration
- End-to-end view of the cloud environment
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, Microsoft Gold Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, and many more.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. Can Object Pooling be applied universally to Unity game objects?
ANS: – Yes, Object Pooling applies to various game objects, including characters, projectiles, enemies, and more.
2. How should object initialization be handled when using Object Pooling?
ANS: – Object initialization can be managed separately within the pooled object script, ensuring proper setup upon activation.
3. Are there scenarios where Object Pooling may not be advantageous?
ANS: – Object Pooling is most beneficial in scenarios involving frequent object instantiation and destruction. In less dynamic scenes, the benefits may be less pronounced.
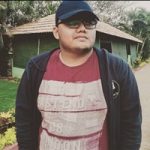
WRITTEN BY Subramanya Datta
Comments