Voiced by Amazon Polly |
Introduction
In Python programming, decorators emerge as a sophisticated tool for enhancing the functionality of functions and methods. They offer an elegant way to modify, extend, or manipulate the behavior of functions without altering their core logic. Understanding the syntax and concept of higher-order functions is crucial to unlocking the full potential of decorators. In this comprehensive guide, we will unravel the intricacies of Python decorators, exploring their syntax, use cases, examples with outputs, pros, cons, and ultimately, how they contribute to cleaner and more efficient code.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Understanding the Basics
Decorators, in essence, are functions that modify other functions or methods. They provide a concise and elegant way to wrap, extend, or manipulate the behavior of functions without altering their core logic. The key to understanding decorators lies in grasping their syntax and the concept of higher-order functions.
Syntax of Decorators
A decorator is a function prefixed with the @ symbol placed above the function definition. Here’s a simple example:
1 2 3 |
@decorator_function def my_function(): # Function logic here |
In this example, decorator_function is the decorator that will be applied to my_function.
Use Cases to Create Own Decorators
- Logging and Timing
Decorators are often employed for logging and timing functions. Let’s create a decorator that logs information about the execution of a function.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
def log_time(func): def wrapper(*args, **kwargs): start_time = time.time() result = func(*args, **kwargs) end_time = time.time() print(f"{func.__name__} executed in {end_time - start_time:.2f} seconds") return result return wrapper @log_time def slow_operation(): time.sleep(2) return "Operation complete" |
Output:
slow_operation executed in 2.00 seconds
2. Authorization and Authentication
Decorators can enforce authorization and authentication checks before executing functions.
1 2 3 4 5 6 7 8 9 10 11 |
def authenticate(func): def wrapper(*args, **kwargs): if is_user_authenticated(): return func(*args, **kwargs) else: raise PermissionError("Authentication failed. User not authorized.") return wrapper @authenticate def sensitive_operation(): return "Sensitive information accessed" |
Output:
PermissionError: Authentication failed. User not authorized.
3. Caching
Decorators can implement caching mechanisms, avoiding redundant computations.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
def memoize(func): cache = {} def wrapper(*args): if args not in cache: cache[args] = func(*args) return cache[args] return wrapper @memoize def fibonacci(n): if n <= 1: return n else: return fibonacci(n - 1) + fibonacci(n - 2) |
Output:
fibonacci(10) executed in 0.00 seconds
4. Chaining Decorators
Python allows chaining multiple decorators, creating a chain of transformations.
In this example, decorator3 is applied first, followed by decorator2, and finally, decorator1.
1 2 3 4 5 |
@decorator1 @decorator2 @decorator3 def my_function(): # Function logic here |
5. Class-Based Decorators
Python supports class-based decorators, where a class implements the __call__ method.
1 2 3 4 5 6 7 8 9 10 11 12 13 |
class MyDecorator: def __init__(self, func): self.func = func def __call__(self, *args, **kwargs): print(f"Decorator is called before {self.func.__name__}") result = self.func(*args, **kwargs) print(f"Decorator is called after {self.func.__name__}") return result @MyDecorator def my_function(): print("Function logic here") |
Output:
Decorator is called before my_function
Function logic here
Decorator is called after my_function
Pros and Cons
Pros
- Modularity: Decorators promote modular code by separating concerns and allowing the addition of functionality without modifying the core logic.
- Readability: Python decorators enhance code readability by isolating common functionality, making the code more concise and focused.
- Reusability: Once defined, decorators can be applied to multiple functions, promoting code reuse and reducing redundancy.
Cons
- Order Dependency: The order in which decorators are applied matters, as they are executed from the innermost to the outermost.
- Debugging Complexity: Overuse of decorators or complex nested decorators can make code harder to debug and understand.
Conclusion
As you delve deeper into the Python ecosystem, mastering the art of decorators becomes a valuable skill. Their simplicity belies their power, making them a key feature for writing clean, efficient, and modular code. Experiment with decorators, explore advanced use cases and witness firsthand how they elevate your Python programming experience.
Drop a query if you have any questions regarding Decorators and we will get back to you quickly.
Empowering organizations to become ‘data driven’ enterprises with our Cloud experts.
- Reduced infrastructure costs
- Timely data-driven decisions
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is the first Indian Company to win the prestigious Microsoft Partner 2024 Award and is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 850k+ professionals in 600+ cloud certifications and completed 500+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, AWS GenAI Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, Amazon ECS Service Delivery Partner, AWS Glue Service Delivery Partner, Amazon Redshift Service Delivery Partner, AWS Control Tower Service Delivery Partner, AWS WAF Service Delivery Partner, Amazon CloudFront Service Delivery Partner, Amazon OpenSearch Service Delivery Partner, AWS DMS Service Delivery Partner, AWS Systems Manager Service Delivery Partner, Amazon RDS Service Delivery Partner, AWS CloudFormation Service Delivery Partner, AWS Config, Amazon EMR and many more.
FAQs
1. How can decorators enhance code readability?
ANS: – Decorators isolate common functionality, making code more concise, focused, and readable.
2. Are decorators reusable in Python?
ANS: – Yes, decorators can be defined once and applied to multiple functions, promoting code reuse and reducing redundancy.
3. Can I apply multiple decorators to a single function in Python?
ANS: – Yes, Python allows you to chain multiple decorators, applying them in a specified order.
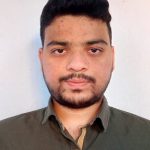
WRITTEN BY Imraan Pattan
Imraan is a Software Developer working with CloudThat Technologies. He has worked on Python Projects using the Flask framework. He is interested in participating in competitive programming challenges and Hackathons. He loves programming and likes to explore different functionalities for creating backend applications.
Comments