Voiced by Amazon Polly |
In modern software development, effective Source Code Management (SCM) plays a critical role in maintaining version control, ensuring smooth collaboration, and streamlining deployment processes. However, alongside SCM, safeguarding secrets (API keys, credentials, tokens) within repositories is equally essential to prevent security vulnerabilities.
This blog covers:
- SCM fundamentals and common branching strategies.
- Protected branches, approvals, and auto-merging workflows.
- Secret management in Git to prevent accidental leaks.
- Hands-on labs to reinforce best practices using Git and GitHub.
By the end of this guide, you will have a clear understanding of efficient SCM workflows and practical ways to secure your repositories.
Enhance Your Productivity with Microsoft Copilot
- Effortless Integration
- AI-Powered Assistance
Part 1: Understanding SCM and Branching Strategies
What is SCM?
SCM refers to tracking and managing changes to source code throughout the development lifecycle. It enables:
- Collaboration among developers.
- Version history and rollback capabilities.
- Better organization of features, fixes, and releases.
Among the many SCM tools available, Git is the most widely used due to its distributed nature, branching capabilities, and extensive support.
Common Branching Strategies
Branching strategies define how teams collaborate on code changes. Some commonly used workflows are:
- Git Flow – A structured model for feature development, releases, and hotfixes.
- GitHub Flow – A lightweight approach based on a single main branch with feature branches.
- GitLab Flow – Integrates Git management with CI/CD pipelines and multiple deployment environments.
Why Use Git Flow Over Other Workflows?
- Clear separation of development stages (features, releases, hotfixes).
- Stable main branches (main and develop).
- Structured feature development for better team collaboration.
- Prevents unstable code from reaching production.
- Allows parallel development of multiple features.
Part 2: Protected Branches, Rules, and Policies
What are Protected Branches?
Protected branches enforce rules to prevent direct modifications. These rules help maintain code quality and security.
Common Protection Rules
- Require Pull Requests (PRs) – Enforces collaboration before merging.
- Enforce Code Reviews – Ensures every change is approved by designated reviewers.
- Require Status Checks – Blocks merging until CI/CD pipelines
Part 3: Implementing Approvals and Auto-Merge
What is Approval and Auto-Merge?
- Approvals ensure that specific team members review code before merging.
- Auto-Merge allows PRs to be automatically merged once predefined checks (approvals, tests) succeed.
These features streamline collaboration and reduce manual intervention in Git workflows.
Part 4: Protecting Secrets in Codebases
What are Secrets?
Secrets refer to sensitive information such as:
- API Keys
- Passwords
- Database Credentials
- SSH Keys
Hardcoding secrets in repositories is a major security risk. Attackers scanning public and private repositories can extract credentials and gain unauthorized access.
Best Practices for Protecting Secrets
- Use environment variables instead of embedding credentials in code.
- Leverage secrets management tools such as AWS Secrets Manager, HashiCorp Vault, and GitHub Secrets.
- Implement automated scanning tools like git-secrets to prevent secret leakage.
Git-Secrets is a tool that detects and prevents the accidental commit of sensitive information. It scans for patterns matching passwords, API keys, and credentials.
What Can Git-Secrets Do?
- Blocks commits containing secrets based on predefined patterns.
- Customizable rules to detect organization-specific credentials.
- Scans entire repositories for sensitive data.
- Supports AWS secret detection out-of-the-box.
Hands-On Labs: Implementation Guide
Pre-requisites:
- System Requirements: Windows, Mac, or Linux
- Tools Needed:
- Git – Install from Git Official Website
- Git Bash – Installed automatically with Git
- Git-Secrets – Security tool for scanning secrets
Lab 1: Setting Up Git Flow
Git Flow is a branching model for Git designed to streamline feature development, releases, and hotfixes. It introduces predefined branch structures and commands to help maintain a robust development workflow.
Step 1: Initialize a Git Repository
Create a new project directory and initialize Git:
1 2 3 4 5 |
mkdir gitflow-demo cd gitflow-demo git init |
Step 2: Verify Git Flow Installation
Recent versions of Git (2.38+ and later) come with Git Flow pre-installed. You can check if Git Flow is already installed by running:
1 |
git flow version |
Step 3: Initialize Git Flow
Run the following command to set up Git Flow in your repository:
1 |
git flow init |
This command will prompt you to define branch names. The default values (press Enter to accept) are:
1 2 3 4 5 6 7 8 9 10 11 |
Branch name for production releases: master Branch name for "next release" development: develop Feature branches? [feature/] Release branches? [release/] Hotfix branches? [hotfix/] Support branches? [support/] |
If you are unsure, use the default settings.
Step 4: Create and Merge a Feature Branch
- Start a new feature branch:
A feature branch is used to develop new functionality before merging it into develop.
1 |
git flow feature start new-feature |
This creates a branch called feature/new-feature from develop.
- Make changes to your code, then stage and commit them:
1 2 3 4 5 |
echo "print('Hello, Git Flow')" > script.py git add . git commit -m "Add new feature" |
- Finish the feature branch (this merges it into develop and deletes the feature branch):
1 |
git flow feature finish new-feature |
Lab 2: Protecting a Branch on GitHub
Step 1: Push Your Repository to GitHub
- Create a new repository on GitHub.
- Link the local repository:
git remote add origin https://github.com/your-username/gitflow-demo.git
- Push the master and develop branches:
1 2 3 |
git push -u origin master git push -u origin develop |
Step 2: Enable Branch Protection
- Go to Settings in your GitHub repository.
- Select Branches from the left menu.
- Click Add Branch Ruleset and enter master as the branch name.
- Enable protections:
- Require pull request reviews before merging
- Require status checks to pass before merging
- Click Save Changes.
Lab 3: Enable Auto-Merge in GitHub
- Go to Settings > General in your GitHub repository.
- Scroll to the Pull Requests section.
- Enable Allow auto-merge.
Step 2: Assign PR Reviewers
- Navigate to Settings > Collaborators & Teams.
- Add team members or assign specific reviewers for PR approvals.
Step 3: Test Auto-Merge
- Create a new feature branch:
1 |
git checkout -b feature-branch |
- Make changes and commit:
1 2 3 4 5 |
echo "print('Hello, Auto-Merge')" > auto_merge.py git add . git commit -m "Add auto-merge test feature" |
- Push the branch to GitHub:
1 |
git push origin feature-branch |
- Open a Pull Request on GitHub:
- Go to Pull Requests > New Pull Request
- Select feature-branch as the source and develop as the target.
- Ensure all required reviews and status checks pass.
- Observe auto-merge in action:
- If all conditions are met, GitHub will automatically merge the PR.
Lab 4: Protecting Secrets with Git-Secrets
Step 1: Install Git-Secrets
For macOS/Linux:
1 |
brew install git-secrets |
For Windows:
1 |
git clone https://github.com/awslabs/git-secrets.git |
1 2 3 |
cd git-secrets make install |
Note: If make install fails, ensure make is installed. You can install it using:
1 |
choco install make |
Step 2: Configure Git-Secrets
Initialize Git-Secrets in a Repository
1 |
git secrets --install |
This installs hooks that prevent commits with secrets.
Register AWS Secret Patterns
1 |
git secrets --register-aws |
This registers common AWS key patterns, such as AWS_SECRET_ACCESS_KEY and AWS_ACCESS_KEY_ID.
Verify Installed Hooks
1 |
ls .git/hooks/ |
You should see pre-commit, commit-msg, and prepare-commit-msg hooks.
Step 3: Test Secret Protection
Create a Test File
1 |
vi test.txt |
Add the following content:
1 2 3 4 5 6 7 |
Testing git secrets! password=ex@mplepassword password=****** More text... |
Save and exit the file.
Add a Custom Pattern to Block Passwords
1 |
git secrets --add 'password\s*=\s*.+' |
This ensures any string containing password = something is blocked.
Allow a Specific False Positive
1 |
git secrets --add --allowed --literal 'ex@mplepassword' |
This allows the specific password ex@mplepassword for testing purposes.
Scan the File for Secrets
1 |
git secrets --scan test.txt |
Expected output:
/tmp/example:3:password=******
[ERROR] Matched prohibited pattern
This means Git-Secrets correctly identified a violation and would block a commit.
Step 4: Prevent Committing Secrets
Try Adding and Committing the File
1 2 3 |
git add test.txt git commit -m "Testing secret detection" |
If git-secrets is working correctly, the commit should be rejected.
Expected output:
[ERROR] Matched prohibited pattern
Commit failed
Bypass Git-Secrets (Not Recommended for Real Use)
If you must commit (e.g., for testing purposes), use:
1 |
git commit -m "Bypassing git-secrets" --no-verify |
Warning: This will allow committing secrets and should only be used in controlled environments.
Step 5: Test Secret Protection
- Create a file containing secrets
Create a file named secrets.txt and add the following content:
1 |
echo "AWS_SECRET_ACCESS_KEY=examplekey123" > secrets.txt |
Verify the content using:
1 |
cat secrets.txt |
- Scan the file for secrets
1 2 3 |
Run git-secrets to check if it detects the secret: git secrets --scan secrets.txt |
Expected output:
secrets.txt:1:AWS_SECRET_ACCESS_KEY=examplekey123
[ERROR] Matched prohibited pattern
- Try Committing the File (It Should Fail!)
Run the following commands to test the pre-commit hook:
1 2 3 |
git add secrets.txt git commit -m "Adding secret" |
Expected output:
[ERROR] Matched prohibited pattern
Commit failed: Detected sensitive information.
This means git-secrets is successfully blocking the commit!
Mastering SCM best practices and secrets management is crucial for efficient and secure software development. By implementing Git Flow, enforcing protected branches, and using security tools like Git-Secrets, you can improve collaboration and reduce security risks.
- Follow branching strategies to structure your development.
- Use approvals and auto-merge for seamless PR management.
- Secure your codebase using Git-Secrets and secrets management tools.
Access to Unlimited* Azure Trainings at the cost of 2 with Azure Mastery Pass
- Microsoft Certified Instructor
- Hands-on Labs
- EMI starting @ INR 4999*
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is the first Indian Company to win the prestigious Microsoft Partner 2024 Award and is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, AWS GenAI Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, Amazon ECS Service Delivery Partner, AWS Glue Service Delivery Partner, Amazon Redshift Service Delivery Partner, AWS Control Tower Service Delivery Partner, AWS WAF Service Delivery Partner, Amazon CloudFront, Amazon OpenSearch, AWS DMS, AWS Systems Manager, Amazon RDS, and many more.
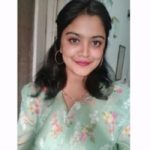
WRITTEN BY Komal Singh
Comments