Voiced by Amazon Polly |
Introduction
When analyzing the performance of algorithms, we often consider their order of growth or how their performance scales as the input size increases. In C#, we can use Big O notation to describe the order of growth of algorithms. Big O notation provides an upper bound on the growth rate of an algorithm’s running time in terms of the input size.
Here are some common orders of growth, ordered from best to worst:
- O(1): constant time – The running time does not depend on the input size. Examples include accessing an array element by index or performing a single arithmetic operation.
- O(log n): logarithmic time – The running time increases slowly as the input size grows. Examples include binary search on a sorted array or searching a balanced binary search tree.
- O(n): linear time – The running time increases proportionally to the input size. Examples include iterating through an array or linked list.
- O(n log n): linearithmic time – The running time increases faster than linear time but slower than quadratic time. Examples include merge sort and quicksort.
- O(n^2): quadratic time – The running time grows exponentially with the input size. Examples include bubble sort and selection sort.
- O(2^n): exponential time – The running time grows extremely fast with the input size. Examples include brute force algorithms that try every possible combination of inputs.
Examples of How to Analyze the Order of Growth of Algorithms in C#
- Iterating through an array
Consider the following code that iterates through an array of size n and performs a constant-time operation on each element:
1 2 3 4 5 |
int[] arr = new int[n]; for (int i = 0; i < n; i++) { Console.WriteLine(arr[i]); } |
- Binary search
Consider the following code that performs a binary search on a sorted array of size n:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 |
int BinarySearch(int[] arr, int key) { int low = 0; int high = arr.Length - 1; while (low <= high) { int mid = (low + high) / 2; if (arr[mid] == key) { return mid; } else if (arr[mid] < key) { low = mid + 1; } else { high = mid - 1; } } return -1; } |
Since each, while loop iteration halves the search space, the running time is O(log n).
- Bubble sort
Consider the following code that performs bubble sort on an array of size n:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
void BubbleSort(int[] arr) { int n = arr.Length; for (int i = 0; i < n - 1; i++) { for (int j = 0; j < n - i - 1; j++) { if (arr[j] > arr[j + 1]) { int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } |
- Quick sort
Consider the following code that performs a quick sort on an array of size n:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
void QuickSort(int[] arr, int left, int right) { if (left < right) { int pivotIndex = Partition(arr, left, right); QuickSort(arr, left, pivotIndex - 1); QuickSort(arr, pivotIndex + 1, right); } } int Partition(int[] arr, int left, int right) { int pivot = arr[right]; int i = left - 1; for (int j = left; j < right; j++) { if (arr[j] < pivot) { i++; int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } int temp2 = arr[i + 1]; arr[i + 1] = arr[right]; arr[right] = temp2; return i + 1; } |
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Conclusion
Since the partition function takes linear time and is called recursively log n times, the running time is O(n log n). In conclusion, when analyzing the performance of algorithms in C#, it is important to consider their order of growth. Big O notation provides a useful way to describe the upper bound on the growth rate of an algorithm’s running time in terms of the input size. We can choose the most efficient algorithm for a given problem by understanding the order of growth of different algorithms.
Making IT Networks Enterprise-ready – Cloud Management Services
- Accelerated cloud migration
- End-to-end view of the cloud environment
About CloudThat
CloudThat is an official AWS (Amazon Web Services) Advanced Consulting Partner and Training partner and Microsoft Gold Partner, helping people develop knowledge of the cloud and help their businesses aim for higher goals using best-in-industry cloud computing practices and expertise. We are on a mission to build a robust cloud computing ecosystem by disseminating knowledge on technological intricacies within the cloud space. Our blogs, webinars, case studies, and white papers enable all the stakeholders in the cloud computing sphere.
Drop a query if you have any questions regarding C#, Algorithms, I will get back to you quickly.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. What is an algorithm's growth order in C#?
ANS: – The order of growth of an algorithm in C# refers to the computational complexity or the rate at which the algorithm’s performance (time or space) scales with the input size. It is commonly expressed using Big O notation, which provides an upper bound on the growth rate. For example, an algorithm with a time complexity of O(n) means that the algorithm’s running time grows linearly with the input size.
2. Can the order of growth of an algorithm be different in C# compared to other programming languages?
ANS: – An algorithm’s growth order is determined by its fundamental characteristics and logic rather than the programming language. Therefore, the order of growth remains the same across different programming languages. However, the actual running times of algorithms can vary due to language-specific optimizations and hardware differences.
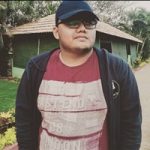
WRITTEN BY Subramanya Datta
Comments