Voiced by Amazon Polly |
Moto Library
Moto is a Python package for unit testing that mimics AWS services. It is an essential tool for developers working with cloud-based apps because it offers a means to mimic AWS infrastructure without accessing AWS via API calls.
Moto is frequently used to test apps that communicate with AWS services, including AWS Lambda, Amazon DynamoDB, Amazon S3, etc. Moto provides safe, dependable, and effective testing environments, allowing developers to test their cloud-dependent code separately.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Why Use Moto?
Testing can be expensive, slow, and sometimes impractical when developing applications that interact with AWS. Here’s why Moto is useful:
- Cost Efficiency: Running real AWS services incurs costs, whereas Moto eliminates unnecessary expenses.
- Faster Testing: Since no actual API calls are made, tests run much faster.
- Offline Testing: Developers can test AWS integrations locally without an internet connection.
- Isolation: Moto helps isolate AWS-related code from the rest of the application, ensuring controlled test environments.
- Ease of Use: It seamlessly integrates with Python’s testing frameworks like unittest and pytest.
Installation
To get started with Moto, you can install it using pip:
1 |
pip install moto |
Example: Mocking Amazon S3
Suppose your application uploads a file to an Amazon S3 bucket. You want to write a test for that without actually uploading the file to AWS. Here’s how you’d do it using Moto:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 |
import mock_s3 import boto3 import pytest @mock_s3 def test_upload_file_to_s3(): s3 = boto3.client('s3', region_name='us-east-1') s3.create_bucket(Bucket='my-test-bucket') s3.put_object(Bucket='my-test-bucket', Key='test.txt', Body='Hello Moto!') response = s3.get_object(Bucket='my-test-bucket', Key='test.txt') content = response['Body'].read().decode('utf-8') assert content == 'Hello Moto!' |
This test creates a virtual Amazon S3 bucket, uploads a file, and checks the contents — all without ever touching the real AWS environment.
How Moto Works Under the Hood?
Moto uses decorators (@mock_s3, @mock_dynamodb, etc.) or context managers (with mock_s3():) to intercept boto3 calls and reroute them to a local, in-memory mock.
It sets up a “fake” backend for each service you are testing, which behaves like the real AWS APIs — respecting permissions, errors, and API patterns. This makes your tests reliable and close to real-world behavior.
Use Cases
Moto is especially useful in the following scenarios:
- CI/CD pipelines: Running tests in a GitHub Actions or Jenkins pipeline without requiring AWS credentials or setting up cloud infrastructure.
- Offline development: Working on AWS integrations offline, using Moto to simulate the services locally.
- Test-driven development (TDD): You can write tests before implementing the AWS logic, then use Moto to simulate the expected AWS behavior.
- Load testing failure handling: Simulate service failures or missing resources (e.g., missing Amazon S3 buckets) to test how your application handles errors.
Moto provides a simple yet powerful way to integrate AWS mocking into your workflow for teams building data pipelines, serverless applications, or cloud-native apps.
Supported AWS Services
Moto supports a wide range of AWS services, including but not limited to:
- Amazon S3 – For bucket and object operations
- Amazon EC2 – For instances, images, and volumes
- Amazon DynamoDB – For table operations
- AWS Lambda – For function creation and invocation
- Amazon SNS/Amazon SQS – For message queuing and pub-sub
Moto’s official documentation contains a comprehensive list of supported services.
Things to Keep in Mind
- Moto is for testing only. Never use it in production environments.
- Not all AWS features are supported. While Moto covers most basic and intermediate use cases, it may not support advanced configurations or newer AWS services.
- Shared state can be tricky. Since Moto uses global mocks, ensure tests are isolated to prevent interference.
Best Practices
- Use fixtures or context managers in pytest to cleanly control the scope of mocks.
- Combine Moto with tools like pytest-mock or freezegun for full testing flexibility.
- Test edge cases like:
- Missing resources (e.g., bucket not found)
- Access denied scenarios
- Conflicting resource creation
- Always pin versions of moto and boto3 in your requirements.txt to avoid compatibility surprises.
Conclusion
Whether you mock Amazon S3 uploads, Amazon DynamoDB tables, or Amazon EC2 instances, Moto lets you test AWS-powered apps with confidence and control.
Drop a query if you have any questions regarding Moto library and we will get back to you quickly.
Making IT Networks Enterprise-ready – Cloud Management Services
- Accelerated cloud migration
- End-to-end view of the cloud environment
About CloudThat
CloudThat is an award-winning company and the first in India to offer cloud training and consulting services worldwide. As a Microsoft Solutions Partner, AWS Advanced Tier Training Partner, and Google Cloud Platform Partner, CloudThat has empowered over 850,000 professionals through 600+ cloud certifications winning global recognition for its training excellence including 20 MCT Trainers in Microsoft’s Global Top 100 and an impressive 12 awards in the last 8 years. CloudThat specializes in Cloud Migration, Data Platforms, DevOps, IoT, and cutting-edge technologies like Gen AI & AI/ML. It has delivered over 500 consulting projects for 250+ organizations in 30+ countries as it continues to empower professionals and enterprises to thrive in the digital-first world.
FAQs
1. What is Moto in Python?
ANS: – Moto is a library for mocking AWS services during testing in Python.
2. Does Moto work with Boto3?
ANS: – Yes, Moto is designed to mock AWS services accessed via the boto3 SDK.
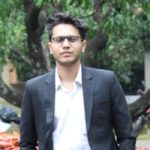
WRITTEN BY Raghavendra Santosh Kulkarni
Raghavendra is a skilled Full Stack Developer with expertise in a wide range of technologies. He has a strong working knowledge of AWS and is always looking to learn about new and emerging technologies. In addition to his technical skills, Raghavendra is a highly motivated and dedicated professional, committed to delivering high quality work.
Comments