Voiced by Amazon Polly |
Overview
Functions are essential for structuring and organizing code in the C programming language. They are discrete code blocks that can be called from other program sections and carry out particular functions. This blog will review the foundations of functions in C, their syntax, and some examples to show how to use them.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Introduction
A function in C is a self-contained chunk of code that carries out a particular task. It is a series of instructions that can be called repeatedly from various program sections. Functions encourage the structuring of code, which facilitates understanding and maintenance.
Function's Anatomy
A C function’s fundamental syntax looks like this:
1 2 3 4 5 6 7 8 9 10 11 |
return_type function_name(parameters) { // Function body // Statements to perform a specific task // Return statement (if applicable) Return value; } |
Now let us study the parts:
- Return Type: The return type of a function indicates the kind of data it will produce. The return type is void if the function returns nothing.
- Function Name: Use the function name as a unique identifier to invoke the function from other program sections.
- Parameters: Acceptable input values for the function. The parenthesis stays empty when a function doesn’t take any input.
- Function Body: The code block surrounded in curly brackets {} is the function body. This is where the function’s real functionality is located.
- Return Statement: The function uses the return statement to supply the value if intended to return a value.
Advantages of using the function
- Typically, a complex issue is broken down into smaller issues before resolution. C uses functions to implement this divide-and-conquer strategy. A program can be broken down into functions, each carrying out a distinct task. Thus, using C functions divides and modularizes a program’s work.
- Using functions helps prevent code duplication when needed repeatedly and in various locations throughout the program.
- The program becomes simple to comprehend, adapt, debug, and test. Writing the program and comprehending the tasks performed by its many components became easier.
- A library allows for the reusability of functions by storing them.
Types of functions in C programming
- Library Functions
- User-defined Functions
Function Example
Here’s how to write a basic function that adds two numbers:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
#include <stdio.h> // Function prototype int add(int x, int y); int main() { int num1 = 5, num2 = 10; int sum = add(num1, num2); printf("Sum of %d and %d is: %d\n", num1, num2, sum); return 0; } // Function definition int add(int x, int y) { return x + y; } |
In this function:
- The add function has two integer parameters (x and y).
- Two integers, num1 and num2, are declared in the main function. These numbers are then passed to the add function, and the outcome is stored in the sum variable.
- Lastly, we use printf to output the outcome.
Prototypes of Function
A function’s return type, name, and parameters are declared in a function prototype before implementation. It permits the function’s structure to be understood by the compiler before its usage in the program. In the previous example, int add(int x, int y); is the prototype function.
The Power of Modularity
A fundamental idea in software development, modularity is encouraged by functions. A program is easier to understand and maintain when divided into smaller sections. The general logic of the program becomes clearer as each function serves a particular purpose.
Look at this basic illustration of a function that determines a number’s square:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 |
#include <stdio.h> // Function prototype int square (int x); int main () { int num = 5; int result = square(num); printf ("Square of %d is: %d\n", num, result); return 0; } // Function definition int square(int x) { return x * x; } |
The logic is segregated into a modular unit, and the main function is made easier to read by enclosing the square computation in a function.
Function Recursion
Recursion is supported by Function C, which enables a function to call itself. Now, let’s build a recursive function to find a number’s factorial:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 |
#include <stdio.h> // Function prototype int factorial (int x); int main() { int num = 5; int result = factorial(num); printf("Factorial of %d is: %d\n", num, result); return 0; } // Recursive function to calculate factorial int factorial (int x) { if (x == 0 || x == 1) { return 1; } else { return x*factorial(x - 1); } } |
In this function, the factorial function keeps calling itself until it reaches the base case (x == 0 or x == 1).
Conclusion
The foundation of C programs are functions that offer modularity and structure. Composing readable and efficient code requires a thorough understanding of its syntax and usage. A C programmer must be proficient in functions, whether writing sophisticated recursive algorithms or basic arithmetic functions.
Drop a query if you have any questions regarding C programming and we will get back to you quickly.
Freedom Month Sale — Discounts That Set You Free!
- Up to 80% OFF AWS Courses
- Up to 30% OFF Microsoft Certs
About CloudThat
CloudThat is an award-winning company and the first in India to offer cloud training and consulting services worldwide. As a Microsoft Solutions Partner, AWS Advanced Tier Training Partner, and Google Cloud Platform Partner, CloudThat has empowered over 850,000 professionals through 600+ cloud certifications winning global recognition for its training excellence including 20 MCT Trainers in Microsoft’s Global Top 100 and an impressive 12 awards in the last 8 years. CloudThat specializes in Cloud Migration, Data Platforms, DevOps, IoT, and cutting-edge technologies like Gen AI & AI/ML. It has delivered over 500 consulting projects for 250+ organizations in 30+ countries as it continues to empower professionals and enterprises to thrive in the digital-first world.
FAQs
1. How is the function declared in C?
ANS: – In C, the function name, parameters, and return type are all included in the function declaration. For example:
1 |
int add(int a, int b); |
2. What is a function prototype used for?
ANS: – A function’s return type, name, and parameters are declared in a function prototype before implementation. This enables correct compilation by allowing the compiler to comprehend the function’s structure before using it in the program.
1 |
int add (int a, int b); //Function prototype |
3. Is it possible for a C function to return more than one value?
ANS: – No, a C function can’t return multiple values at once. Nevertheless, you can accomplish a similar result by using structures or pointers. For example, a function can return more than one value by changing the values that its parameters point to. void getValues(int *result1, int *result2);
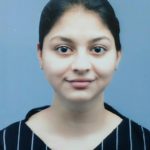
WRITTEN BY Vaishali Bhawsar
Vaishali is working as a Research Associate in CloudThat Technologies. She has good knowledge of Networking, Linux systems & C language, and currently working on various AWS projects along with, Terraform, Docker, and Ansible. She enjoys painting and cooking during her free time.
Comments