Overview
In today’s world of concurrent programming, writing thread-safe programs is paramount to ensure data integrity and prevent race conditions. Python, with its rich ecosystem and support for threading, provides developers with powerful tools to create multi-threaded applications. However, threading in Python comes with its challenges, especially when it comes to ensuring thread safety. In this guide, we will delve into the best practices for writing thread-safe programs in Python.
Thread Safety
Before diving into the techniques for writing thread-safe programs, it’s crucial to understand what thread safety means.
Achieving thread safety involves proper synchronization mechanisms to ensure that shared resources are accessed and modified in a controlled and consistent manner.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Utilizing Locks
One of the most common synchronization mechanisms in Python is locks. A lock is a synchronization primitive that allows only one thread to execute a particular code section at a time. Python provides locks through the threading.Lock
class, which can be used to protect critical sections of code from concurrent access.
1 2 3 4 5 6 7 8 9 10 |
```python import threading lock = threading.Lock() def thread_safe_function(): with lock: # Critical section of code # Access and modify shared resources safely ``` |
By utilizing locks, developers can enforce exclusive access to shared resources, ensuring that only one thread can modify them at any time, thereby preventing race conditions.
Using Thread-Safe Data Structures
Python offers several built-in thread-safe data structures in its collections
module, such as Queue
, Deque
, and Counter
. These data structures are designed to be accessed safely from multiple threads without external synchronization mechanisms.
1 2 3 4 5 6 7 8 9 10 11 |
```python from collections import deque thread_safe_deque = deque() # Thread 1 thread_safe_deque.append(1) # Thread 2 element = thread_safe_deque.pop() ``` |
By leveraging thread-safe data structures, developers can avoid the complexities associated with manual synchronization using locks and achieve thread safety more conveniently.
Atomic Operations with Atomicity
Atomicity is another crucial aspect of writing thread-safe programs. An operation is atomic if it occurs instantaneously from the perspective of other threads. In Python, certain operations, such as incrementing or decrementing variables, may not be inherently atomic. To ensure atomicity, developers can use the threading
module’s Lock
or RLock
(recursive lock) in conjunction with these operations.
1 2 3 4 5 6 7 8 9 10 11 12 |
```python class Counter: def __init__(self): self.value = 0 self.lock = threading.Lock() def increment(self): with self.lock: self.value += 1 counter = Counter() ``` |
By encapsulating non-atomic operations within a lock, developers can ensure that these operations are performed atomically, thus maintaining thread safety.
Testing Thread Safety
Testing is a crucial aspect of writing thread-safe programs. Developers should thoroughly test their multi-threaded applications under various concurrency scenarios to identify and address potential race conditions and synchronization issues. Tools such as unittest
and pytest
can write and execute unit tests for thread safety.
Conclusion
Writing thread-safe programs in Python requires a solid understanding of concurrency concepts and synchronization mechanisms. By leveraging locks, thread-safe data structures, atomic operations, and minimizing shared mutable state, developers can ensure that their multi-threaded applications are free from race conditions and other concurrency-related bugs. Additionally, thorough testing is essential to validate the correctness and robustness of thread-safe programs. With careful design and implementation, Python developers can create reliable and efficient concurrent applications that scale to meet the demands of modern computing environments.
Drop a query if you have any questions regarding Python and we will get back to you quickly.
Making IT Networks Enterprise-ready – Cloud Management Services
- Accelerated cloud migration
- End-to-end view of the cloud environment
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, and many more.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. What are race conditions, and how do they affect Python's thread safety?
ANS: – Race conditions occur when the outcome of concurrent operations depends on the timing or interleaving of threads. In Python, they can lead to unpredictable behavior and data corruption. Employing synchronization mechanisms like locks or thread-safe data structures helps mitigate race conditions and ensures thread safety.
2. How can I determine if my Python program is thread-safe?
ANS: – Thorough testing and analysis under various concurrency scenarios are essential. Pay attention to race conditions, data inconsistencies, and deadlock situations. Techniques such as unit testing, code review, and static analysis tools aid in identifying and addressing thread safety issues early in development.
3. Are there performance implications of using locks and synchronization mechanisms in Python?
ANS: – While locks and synchronization mechanisms are crucial for thread safety, they can introduce overhead and potentially impact performance, especially in highly concurrent applications. Strive to balance thread safety and performance by optimizing critical code sections and minimizing lock contention. Performance profiling and benchmarking assist in identifying bottlenecks for better scalability and efficiency.
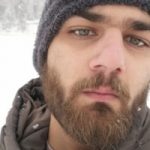
WRITTEN BY Mohmmad Shahnawaz Ahangar
Shahnawaz is a Research Associate at CloudThat. He is certified as a Microsoft Azure Administrator. He has experience working on Data Analytics, Machine Learning, and AI project migrations on the cloud for clients from various industry domains. He is interested to learn new technologies and write blogs on advanced tech topics.
Comments