Voiced by Amazon Polly |
Overview
Reflection, in the context of programming, refers to the ability of a program to inspect, analyze, and even modify its structure and behavior during runtime. In Python, reflection is a powerful tool that allows dynamic interaction with objects, functions, and classes. This feature makes Python an incredibly flexible and expressive language, allowing developers to write more generalized, reusable, and dynamic code.
In this blog post, we will explore how reflection works in Python, its core concepts, and how to use it effectively. By the end, you will have a deeper understanding of reflection, its use cases, and how it can make your Python programming more dynamic and flexible.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Reflection in Python
Reflection in Python refers to the ability of a program to examine the types or properties of objects at runtime rather than compile-time. This includes querying an object’s attributes methods or changing the object’s structure on the fly.
Reflection enables you to dynamically inspect or manipulate the Python objects during the program execution, which is generally impossible in statically typed languages like Java or C++. Python’s dynamic nature allows reflection to play a significant role in its ecosystem.
Core Features of Reflection in Python
- Inspecting Object Properties: Reflection lets you retrieve information about an object’s properties or metadata (such as its methods, attributes, or class).
- Dynamic Object Creation: Reflection allows the dynamic creation of new classes or objects during runtime.
- Modifying Objects: You can modify attributes or methods of an object or class at runtime.
- Accessing Callable Objects: Reflection enables you to access functions or methods dynamically, whether they belong to an object or class.
Python's Reflection Mechanisms
Python provides different built-in functions and modules that allow you to leverage reflection. Let us look at some of the most commonly used tools and techniques.
- The getattr() Function
One of the most commonly used reflection functions in Python is getattr(). This function allows you to access an object’s attributes or methods dynamically. If the attribute or method does not exist, it can raise an AttributeError, but you can also specify a default value.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class MyClass: def __init__(self, name): self.name = name def greet(self): return f"Hello, my name is {self.name}" obj = MyClass("abc") # Using getattr to access the attribute dynamically name = getattr(obj, 'name', 'Unknown') print(name) # Output: abc # Using getattr to access a method dynamically greeting = getattr(obj, 'greet')() print(greeting) # Output: Hello, my name is abc |
- The setattr() Function
The setattr() function dynamically allows you to set or modify an object’s attribute during runtime.
1 2 3 4 5 6 7 8 9 10 11 12 |
class Person: def __init__(self, name): self.name = name # Creating an object of Person person = Person("abc") # Using setattr to dynamically modify the attribute setattr(person, 'name', xyz) # Output the modified attribute print(person.name) # Output: xyz |
- The hasattr() Function
Before accessing an attribute or method, checking if it exists is good practice. Python provides the hasattr() function, which returns True if the object has the specified attribute or method and False otherwise.
1 2 3 4 |
if hasattr(person, 'name'): print("Name attribute exists.") else: print("Name attribute does not exist.") |
- The delattr() Function
Reflection also allows you to delete attributes of objects dynamically using delattr(). This can be useful in various scenarios, such as cleaning up resources.
1 2 3 4 5 6 |
# Deleting the 'name' attribute dynamically delattr(person, 'name') # Checking if 'name' exists after deletion if not hasattr(person, 'name'): print("Name attribute deleted.") # Output: Name attribute deleted. |
- The type() Function
Another reflection-related function is type(). This built-in function allows you to check the type of an object, which can be particularly useful for debugging or for conditional logic based on the type of an object.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
x = 42 print(type(x)) # Output: <class 'int'> y = "Hello" print(type(y)) # Output: <class 'str'> We can also dynamically use type() to create new classes at runtime. # Dynamically creating a class MyClass = type('MyDynamicClass', (object,), {'x': 5}) # Creating an instance of the newly created class obj = MyClass() print(obj.x) # Output: 5 print(type(obj)) # Output: <class '__main__.MyDynamicClass'> |
- The inspect Module
Python’s inspect module offers a richer set of reflection tools for working with live objects, including functions and classes. It allows you to inspect the attributes, parameters, and docstrings of functions or methods.
1 2 3 4 5 6 7 8 9 10 11 |
import inspect def my_function(a, b): """This is a sample function.""" return a + b # Inspecting the function's signature print(inspect.signature(my_function)) # Output: (a, b) # Inspecting the function's docstring print(inspect.getdoc(my_function)) # Output: This is a sample function. |
- The globals() and locals() Functions
Reflection also extends to the global and local scopes. The globals() function returns a dictionary of the current global symbol table, and locals() returns a dictionary of the current local symbol table.
1 2 3 4 5 6 7 |
x = 10 print(globals()) # Output: {'x': 10, ...} def test(): y = 20 print(locals()) test() # Output: {'y': 20} |
You can manipulate these dictionaries to change variables in different scopes at runtime.
Practical Use Cases of Reflection in Python
- Dynamic Method Invocation
Reflection is useful when you want to call methods on an object dynamically. This is common in scenarios like plugin systems, where the execution methods are determined at runtime.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
class Calculator: def add(self, a, b): return a + b def subtract(self, a, b): return a - b def invoke_method(obj, method_name, *args): method = getattr(obj, method_name) return method(*args) calc = Calculator() result = invoke_method(calc, 'add', 5, 3) print(result) # Output: 8 |
- Frameworks and Libraries
Many frameworks, including web frameworks like Django or Flask, use reflection to introspect models, controllers, or routes dynamically. This is essential for automatic route registration or dependency injection systems.
- Serialization and Deserialization
Reflection can be used in serialization and deserialization libraries, such as JSON or XML parsers, where the data structure is dynamically examined, and objects are created or modified based on the data.
- Unit Testing
Reflection allows the program to discover test methods, attributes, or resources in testing frameworks, making it easier to run tests dynamically.
Conclusion
Understanding how to leverage reflection can help you in tasks like creating dynamic plugins, building flexible libraries, and even performing advanced debugging.
By using reflection wisely and in moderation, you can make your Python code more versatile and capable of handling real-time changes or decisions, pushing your programming skills to a new level.
Drop a query if you have any questions regarding Reflection in Python and we will get back to you quickly.
Empowering organizations to become ‘data driven’ enterprises with our Cloud experts.
- Reduced infrastructure costs
- Timely data-driven decisions
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is the first Indian Company to win the prestigious Microsoft Partner 2024 Award and is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, Microsoft Gold Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, AWS GenAI Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, Amazon ECS Service Delivery Partner, AWS Glue Service Delivery Partner, Amazon Redshift Service Delivery Partner, AWS Control Tower Service Delivery Partner, AWS WAF Service Delivery Partner, Amazon CloudFront Service Delivery Partner, Amazon OpenSearch Service Delivery Partner, AWS DMS Service Delivery Partner, AWS Systems Manager Service Delivery Partner, Amazon RDS Service Delivery Partner, AWS CloudFormation Service Delivery Partner and many more.
FAQs
1. What is Reflection in Python?
ANS: – Reflection in Python refers to the ability of a program to examine and modify its structure and behavior at runtime. This includes tasks such as inspecting classes, functions, and methods and dynamically invoking functions or accessing attributes. Python provides several built-in functions like getattr(), setattr(), hasattr(), and dir() to perform reflection-based tasks.
2. How can I dynamically use Reflection to call a function in Python?
ANS: – You can use reflection to dynamically call a function in Python by using the getattr() function, which allows you to access an attribute (such as a method) of an object by name. Here’s an example:
1 2 3 4 5 6 7 8 |
class MyClass: def greet(self): return "Hello, World!" obj = MyClass() method_name = "greet" method = getattr(obj, method_name) # Access method dynamically print(method()) # Calls greet() method |
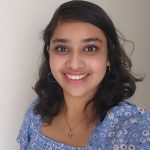
WRITTEN BY Hridya Hari
Hridya Hari works as a Research Associate - Data and AIoT at CloudThat. She is a data science aspirant who is also passionate about cloud technologies. Her expertise also includes Exploratory Data Analysis.
Comments