Voiced by Amazon Polly |
Overview
Object-Oriented Programming (OOP) is a programming paradigm that has transformed how we design and build software. C#, as a powerful and versatile language, fully embraces the principles of OOP. In this blog post, we’ll delve into the essential OOP concepts in C#, understanding how they contribute to creating robust, maintainable, and scalable software.
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Introduction
Classes and Objects: The Building Blocks
At the core of OOP are classes and objects. In C#, a class serves as a blueprint for creating objects. It defines the attributes (data members) and methods (functions) that objects of that class will possess. Objects, however, are instances of classes, encapsulating data and behavior in a cohesive unit.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
class Car { // Fields (attributes) public string Model; public int Year; // Methods (behavior) public void Start() { Console.WriteLine("Engine started."); } } Car myCar = new Car(); myCar.Model = "Toyota"; myCar.Year = 2023; myCar.Start(); |
In this example, Car is a class, and myCar is an instance (object) of that class.
Encapsulation: Data Protection and Control
Encapsulation is a fundamental OOP principle that involves bundling data (attributes) and the methods (functions) that operate on that data into a single unit, i.e., a class. It restricts direct access to certain components of an object, ensuring that data remains protected and modifications are controlled.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
class BankAccount { private double balance; public void Deposit(double amount) { // Encapsulation enforces rules for modifying the balance. if (amount > 0) balance += amount; } public double GetBalance() { return balance; } } |
In this case, the balance field is private, and access to it is only permitted through public methods.
Inheritance: Building on Existing Classes
Inheritance allows for creating a new class (derived or child class) based on an existing class (base or parent class). The derived class inherits attributes and behaviors from the base class and can also introduce new ones or modify existing ones.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
class Animal{ public void Eat() { Console.WriteLine("Animal is eating."); } } class Dog : Animal { public void Bark() { Console.WriteLine("Dog is barking."); } } Dog myDog = new Dog(); myDog.Eat(); // Inherited from Animal class myDog.Bark(); // Defined in Dog class |
In this example, Dog inherits the Eat method from the Animal class and adds its Bark method.
Polymorphism: Handling Different Types
Polymorphism allows objects of different classes to be treated as objects of a common base class. This facilitates writing code that works with objects of various derived classes without knowing their specific types.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
class Shape { public virtual void Draw() { Console.WriteLine("Drawing a shape."); } } class Circle : Shape { public override void Draw() { Console.WriteLine("Drawing a circle."); } } Shape myShape = new Circle(); myShape.Draw(); // Calls the Circle class's Draw method |
In this scenario, myShape is of type Shape, yet it represents an instance of Circle. Polymorphism ensures that the appropriate Draw method is invoked at runtime.
Abstraction: Simplifying Complex Systems
Abstraction involves concealing intricate implementation details and exposing only the essential features of an object. In C#, this is achieved using abstract classes and interfaces. Abstract classes cannot be instantiated and may include abstract methods that derived classes must implement.
Example:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
abstract class Shape { public abstract double CalculateArea(); } class Circle : Shape { public double Radius { get; set; } public override double CalculateArea() { return Math.PI * Radius * Radius; } } |
Here, Shape is an abstract class with an abstract method, CalculateArea(). The Circle class inherits from Shape and implements CalculateArea().
Conclusion
A solid grasp of these fundamental OOPs concepts—classes, objects, encapsulation, inheritance, polymorphism, and abstraction—is indispensable when crafting well-structured, maintainable, and extensible C# code. By applying these principles, developers can create organized, efficient, and adaptable software, making the journey in C# development more productive and gratifying.
Drop a query if you have any questions regarding OOPs Concept in C# and we will get back to you quickly.
Making IT Networks Enterprise-ready – Cloud Management Services
- Accelerated cloud migration
- End-to-end view of the cloud environment
About CloudThat
CloudThat is an official AWS (Amazon Web Services) Advanced Consulting Partner and Training partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, Amazon QuickSight Service Delivery Partner, AWS EKS Service Delivery Partner, and Microsoft Gold Partner, helping people develop knowledge of the cloud and help their businesses aim for higher goals using best-in-industry cloud computing practices and expertise. We are on a mission to build a robust cloud computing ecosystem by disseminating knowledge on technological intricacies within the cloud space. Our blogs, webinars, case studies, and white papers enable all the stakeholders in the cloud computing sphere.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. What is the main advantage of using OOPs concepts in C# development?
ANS: – The primary advantage of utilizing OOPs concepts in C# development is the ability to create more organized, maintainable, and extensible software. By structuring code into classes and objects, encapsulating data and behavior, and employing principles like inheritance, polymorphism, and encapsulation, developers can build complex systems with greater ease. OOPs promotes code reusability, reduces complexity, and facilitates collaboration among team members. It also aligns well with real-world problem-solving, making it a powerful approach for modeling and solving complex problems in a structured and efficient manner.
2.
ANS: –
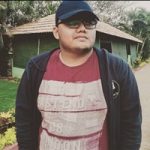
WRITTEN BY Subramanya Datta
Comments