Voiced by Amazon Polly |
Overview
The AWS SDK for Java makes it easier to use AWS Services by giving Java developers access to a collection of consistent and well-known libraries. It helps with considerations related to the API lifecycle, such as credential administration, data marshalling, retries, and serialization. For streamlined development, the AWS SDK for Java enables higher-level abstractions.
The popular AWS service that provides a cloud-based integrated development environment (IDE)for writing, running, and debugging code is AWS Cloud9. It’s simple for you to launch new initiatives with AWS Cloud9. Java, Node.js, JavaScript, Python, PHP, Ruby, Go, and C++ are just a few of more than 40 programming languages already pre-packaged into Cloud9’s working environment. With this launch, we can eliminate the need to invest time installing or configuring software on our work machine. When you operate your Cloud9 development environment on a managed EC2 instance, you receive exclusive sudo rights and a pre-authenticated AWS CLI. Removing the need to install or configure files, SDKs, and plug-ins for your development machine allows you to begin writing code for well-known application stacks within minutes. You can maintain multiple development environments to separate the resources for your project because Cloud9 is cloud-based.
A step-by-step guide to running Java code in an AWS Cloud9 development environment- Part-1
- Create an environment with EC2 with instant type t3.small.
- Open the Integrated Development Environment (IDE) the following screen depicts.
- Now, to check if you have OpenJDK installed or not, check by executing the following command.
$ java -version
If you see the versions, that means you have OpenJDK installed. - In this step, add code by creating a file with the following code and save the File with the name hello.java in the AWS Cloud9 IDE. (To create a file, go to the menu bar, then choose File> New File. To save the File, choose File>Save.)
public class hello {
public static void main(String []args) {
System.out.println(“Hello, World!”);
System.out.println(“The sum of 2 and 3 is 5.”);
int sum = Integer.parseInt(args[0]) + Integer.parseInt(args[1]);
System.out.format(“The sum of %s and %s is %s.\n”,
args[0], args[1], Integer.toString(sum));
}
}
Customized Cloud Solutions to Drive your Business Success
- Cloud Migration
- Devops
- AIML & IoT
A step-by-step guide to running Java code in an AWS Cloud9 development environment- Part-2
- Build and run the code- To convert the hello.java file into a hello.class file, use the Java compiler’s command-line interface. Run the Java compiler with the hello.java file specified in the same directory as the hello.java file using the terminal in the AWS Cloud9 IDE.
$ javac hello.java
- Run the hello.class file using the Java runner’s command-line interface. To accomplish this, launch the Java runner from the same directory as the hello.class file and enter two integers to add along with the name of the hello class defined in the hello.java file. (For example, 5 and 9).
$ java hello 5 9
Output is shown below.
- Now set up to use AWS SDK-In this step, you install Apache Maven in your environment. Maven is a well-known build automation solution that Java projects can use. Maven can be used to create a new Java project after installation. You reference the AWS SDK for Java in this new project. With the help of this AWS SDK for Java, you can easily connect Java code to AWS services like Amazon S3.
- i. Setup Maven
To check whether you have Maven installed or not, use this command-$ mvn -versionif it shows the version, it is installed, and if it shows that the mvn command is not found, it’s not installed.
As it is already installed here so you will get the following output screen.
If it is not installed, use these commands to install Maven if you have Amazon Linux.$ sudo wget http://repos.fedorapeople.org/repos/dchen/apache-maven/epel-apache-maven.repo -O /etc/yum.repos.d/epel-apache-maven.repo
$ sudo sed -i s/\
$releasever/6/g /etc/yum.repos.d/epel-apache-maven.repo
$ sudo yum install -y apache-mavenAnd then again, use the same command to check whether it is installed successfully or not.
$ mvn -versionUse Maven to generate a new Java project. Run the following command in the terminal from the location where you want Maven to create the project to accomplish this. (For example, the root directory of your environment).
mvn archetype:generate -DgroupId=com.mycompany.app -DartifactId=my-app -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=falseThe project in your environment is created with the command mentioned above with the directory layout shown below.
ii. Add AWS SDK code.
This step involves adding code to create an Amazon S3 bucket, display your available buckets, and then delete the bucket just created.
From the Environment window, open the
my app/src/main/java/com/mycompany/app/App.java file.
Replace the File’s current contents with the following code in the editor, then save the App.java file.package com.mycompany.app;
import com.amazonaws.auth.profile.ProfileCredentialsProvider;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
import com.amazonaws.services.s3.model.AmazonS3Exception;
import com.amazonaws.services.s3.model.Bucket;
import com.amazonaws.services.s3.model.CreateBucketRequest;
import java.util.List;
public class App {
private static AmazonS3 s3;
public static void main(String[] args) {
if (args.length < 2) {
System.out.format(“Usage: \n” +
“Example: my-test-bucket us-east-2\n”);
return;
}
String bucket_name = args[0];
String region = args[1];
s3 = AmazonS3ClientBuilder.standard()
.withCredentials(new ProfileCredentialsProvider())
.withRegion(region)
.build();
// List current buckets.
ListMyBuckets();
// Create the bucket.
if (s3.doesBucketExistV2(bucket_name)) {
System.out.format(“\nCannot create the bucket. \n” +
“A bucket named ‘%s’ already exists.”, bucket_name);
return;
} else {
try {
System.out.format(“\nCreating a new bucket named ‘%s’…\n\n”, bucket_name);
s3.createBucket(new CreateBucketRequest(bucket_name, region));
} catch (AmazonS3Exception e) {
System.err.println(e.getErrorMessage());
}
}
// To confirm that the bucket was created.
ListMyBuckets();
// Delete the bucket.
try {
System.out.format(“\nDeleting the bucket named ‘%s’…\n\n”, bucket_name);
s3.deleteBucket(bucket_name);
} catch (AmazonS3Exception e) {
System.err.println(e.getErrorMessage());
}
// To confirm that the bucket was deleted.
ListMyBuckets();
}
private static void ListMyBuckets() {
List buckets = s3.listBuckets();
System.out.println(“My buckets now are:”);
for (Bucket b : buckets) {
System.out.println(b.getName());
}
}
}iii. Build and run the AWS SDK code.
Run the following commands from the terminal to run the code from the previous step. Maven is used by these commands to create an executable JAR file for the project and then to run the JAR using the Java runner. The name of the Amazon S3 bucket and the ID of the AWS Region (here, as an example, my-test-bucket and us-east-2) is used to run with the JAR to create the bucket as input.
For Maven, run the following commands.$ cd my-app
$ mvn packageThe output will be the following screens.
- Now run the following command.
$ java -cp target/my-app-1.0-SNAPSHOT-jar-with-dependencies.jar com.mycompany.app.App my-test-bucket-vls us-east-2
Your results will be the following output.
Get your new hires billable within 1-60 days. Experience our Capability Development Framework today.
- Cloud Training
- Customized Training
- Experiential Learning
About CloudThat
CloudThat, incepted in 2012, is the first Indian organization to offer Cloud training and consultancy for mid-market and enterprise clients. Our business aims to provide global services on Cloud Engineering, Training, and Expert Line. Our expertise in all major cloud platforms, including Microsoft Azure, Amazon Web Services (AWS), VMware, and Google Cloud Platform (GCP), positions us as pioneers.
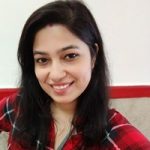
WRITTEN BY Vijaya Lakshmi Singh
Comments