Introduction
Sorting is a common operation in computer science and programming. It involves arranging items in a specific order, usually ascending or descending. This blog post will delve into several well-known sorting algorithms in C#, highlighting their unique characteristics and drawbacks.
Bubble Sort
Bubble sort is a straightforward sorting algorithm that iterates through a list, comparing adjacent elements and swapping them if they are in the incorrect order. This process is repeated until the list is completely sorted. Below is the C# code implementation for bubble sort:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 |
public static void BubbleSort(int[] arr) { int n = arr.Length; for (int i = 0; i < n - 1; i++) { for (int j = 0; j < n - i - 1; j++) { if (arr[j] > arr[j + 1]) { int temp = arr[j]; arr[j] = arr[j + 1]; arr[j + 1] = temp; } } } } |
Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
Selection Sort
Selection sort is a comparison-based sorting algorithm that operates in place. It partitions the input list into two sections: the left end represents the sorted portion, initially empty, while the right end denotes the unsorted portion of the entire list. The algorithm works by locating the smallest element within the unsorted section and swapping it with the leftmost unsorted element, progressively expanding the sorted region by one element. The following is the C# code implementation for the selection sort:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 |
public static void SelectionSort(int[] arr) { int n = arr.Length; for (int i = 0; i < n - 1; i++) { int minIndex = i; for (int j = i + 1; j < n; j++) { if (arr[j] < arr[minIndex]) { minIndex = j; } } int temp = arr[i]; arr[i] = arr[minIndex]; arr[minIndex] = temp; } } |
Insertion Sort
Insertion sort is a basic sorting algorithm that constructs the sorted array gradually, one item at a time. It is less efficient than more advanced algorithms like quicksort, heapsort, or merge sort, especially for large lists. The algorithm operates by sequentially traversing an array from left to right, comparing adjacent elements, and performing swaps if they are out of order. Below is the C# code implementation for insertion sort:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 |
public static void InsertionSort(int[] arr) { int n = arr.Length; for (int i = 1; i < n; i++) { int key = arr[i]; int j = i - 1; while (j >= 0 && arr[j] > key) { arr[j + 1] = arr[j]; j--; } arr[j + 1] = key; } } |
Quick Sort
Quicksort is a sorting algorithm based on the divide-and-conquer approach. It begins by choosing a pivot element from the array and divides the remaining elements into two sub-arrays based on whether they are smaller or larger than the pivot. These sub-arrays are then recursively sorted. Here is the C# code implementation for Quicksort:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 |
public static void QuickSort(int[] arr, int left, int right) { if (left < right) { int pivotIndex = Partition(arr, left, right); QuickSort(arr, left, pivotIndex - 1); QuickSort(arr, pivotIndex + 1, right); } } private static int Partition(int[] arr, int left, int right) { int pivot = arr[right]; int i = left - 1; for (int j = left; j < right; j++) { if (arr[j] < pivot) { i++; int temp = arr[i]; arr[i] = arr[j]; arr[j] = temp; } } int temp2 = arr[i + 1]; arr[i + 1] = arr[right]; arr[right] = temp2; return i + 1; } |
Merge Sort
Merge sort is a sorting algorithm based on the divide-and-conquer principle. It begins by dividing an array into two halves, recursively applying itself to each half, and then merging the two sorted halves back together. The merge operation plays a crucial role in this algorithm
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 |
public static void MergeSort(int[] arr, int left, int right) { if (left < right) { int middle = (left + right) / 2; MergeSort(arr, left, middle); MergeSort(arr, middle + 1, right); Merge(arr, left, middle, right); } } private static void Merge(int[] arr, int left, int middle, int right) { int[] temp = new int[arr.Length]; for (int i = left; i <= right; i++) { temp[i] = arr[i]; } int j = left; int k = middle + 1; int l = left; while (j <= middle && k <= right) { if (temp[j] <= temp[k]) { arr[l] = temp[j]; j++; } else { arr[l] = temp[k]; k++; } l++; } while (j <= middle) { arr[l] = temp[j]; l++; j++; } } |
Conclusion
Each algorithm has advantages and limitations, and the optimal choice depends on the specific needs and constraints of the problem. By understanding the characteristics and behaviors of these sorting algorithms, developers can make informed decisions on which to employ to achieve the desired sorting outcome efficiently.
Making IT Networks Enterprise-ready – Cloud Management Services
- Accelerated cloud migration
- End-to-end view of the cloud environment
About CloudThat
CloudThat is an official AWS (Amazon Web Services) Advanced Consulting Partner and Training partner and Microsoft Gold Partner, helping people develop knowledge of the cloud and help their businesses aim for higher goals using best-in-industry cloud computing practices and expertise. We are on a mission to build a robust cloud computing ecosystem by disseminating knowledge on technological intricacies within the cloud space. Our blogs, webinars, case studies, and white papers enable all the stakeholders in the cloud computing sphere.
Drop a query if you have any questions regarding Sorting Algorithm, I will get back to you quickly.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. What are sorting algorithms in C#?
ANS: – Sorting algorithms in C# arrange elements in a specific order, such as ascending or descending, based on a certain key or comparison criteria. These algorithms take an unordered collection of elements as input and return the same collection with its elements rearranged in the desired order.
2. Can I implement my sorting algorithm in C#?
ANS: – Yes, you can implement your sorting algorithm in C#. Sorting algorithms are often taught in computer science courses, and numerous resources and tutorials are available online that explain the implementation details of various sorting algorithms. By understanding the algorithm’s logic and using C#’s array manipulation and comparison features, you can write your sorting algorithm tailored to your specific requirements.
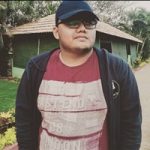
WRITTEN BY Subramanya Datta
Comments