Introduction
A key component of Python programming is exception handling, which enables programmers to handle errors and unexpected events with grace. We will go over the foundations of Python exception handling in this blog post, as well as how it functions and how to write dependable code.
Understanding Exceptions
TypeError
, ValueError
, FileNotFoundError
, and ZeroDivisionError
are examples of common exceptions.Pioneers in Cloud Consulting & Migration Services
- Reduced infrastructural costs
- Accelerated application deployment
The try except Block
The try-except block is Python’s primary mechanism for dealing with exceptions. With this structure, you can declare how to handle an exception in an associated except block and wrap code that might raise one in a try block. Here is an easy example:
1 2 3 4 |
try: result = 10 / 0 # Attempting to divide by zero except ZeroDivisionError: print("Error: Cannot divide by zero.") |
In this example, a ZeroDivisionError is caught, preventing the program from crashing. Instead, the set error message is displayed.
Multiple Exceptions and Generic Handling
Several except blocks or a single block that handles numerous exceptions can be used to handle multiple exceptions. A more general except block can also be used to capture any exception that isn’t specifically handled.
1 2 3 4 5 6 7 8 |
try: # Some code that might raise exceptions except ValueError as ve: print(f"ValueError: {ve}") except TypeError as te: print(f"TypeError: {te}") except Exception as e: print(f"Unexpected error: {e}") |
You can capture the exception instance for additional logging or analysis by using the “as” keyword. However, it’s necessary to utilize caution while using this functionality, as capturing too many exceptions can hide flaws and complicate troubleshooting.
The else and finally Blocks
Python provides else and finally blocks in addition to try and except.
- else block: If there are no exceptions raised by the try block, code inside the else block is executed.
1 2 3 4 5 6 |
try: result = 10 / 2 except ZeroDivisionError: print("Error: Cannot divide by zero.") else: print(f"Result: {result}") |
- finally block: Finally block code runs regardless of whether an exception is raised. It is frequently used for cleanup operations such as closing files or releasing resources.
1 2 3 4 5 6 7 |
try: file = open("example.txt", "r") # Some code that might raise exceptions except FileNotFoundError: print("Error: File not found.") finally: file.close() |
Custom Exceptions
Although Python comes with many built-in exceptions, you can also make your own custom exceptions to stand in for particular fault scenarios in your programs. The base Exception class is where custom exceptions should be derived from.
1 2 3 4 5 6 7 8 |
class CustomError(Exception): def __init__(self, message): super().__init__(message) try: raise CustomError("This is a custom exception.") except CustomError as ce: print(f"CustomError: {ce}") |
You can customize error handling to your application’s particular requirements and give developers debugging code more useful information by generating custom exceptions.
Best Practices for Exception Handling
- Specificity Matters: Be precise when defining exceptions. Only identify and deal with the exceptions you expect. Avoid catching too many exceptions.
- Keep It Simple: Exception-handling code needs to be clear and easy to understand. Keep it free of needless logic.
- Logging and Documentation: To gather information about exceptions for a later examination, use logging. Additionally, document your code so that future developers will understand it.
- Handle Exceptions Locally: Handle exceptions to the point where they arise whenever possible. This makes the code easier to read and facilitates tracing and problem-solving.
- Avoid bare “except”: Bare except statements should be avoided since they catch all exceptions and may have unintended consequences. Always be specific about the exceptions you want to deal with.
Conclusion
One of the most important skills for building reliable and maintainable code in Python is exception handling. By understanding the try except block, handling multiple exceptions, utilizing else and finally blocks, creating custom exceptions, and embracing best practices, you empower yourself to write code that gracefully handles errors and ensures a smoother user experience. Exception handling is not just about preventing crashes; it’s about building resilient and reliable software.
Drop a query if you have any questions regarding Python and we will get back to you quickly.
Empowering organizations to become ‘data driven’ enterprises with our Cloud experts.
- Reduced infrastructure costs
- Timely data-driven decisions
About CloudThat
CloudThat is a leading provider of Cloud Training and Consulting services with a global presence in India, the USA, Asia, Europe, and Africa. Specializing in AWS, Microsoft Azure, GCP, VMware, Databricks, and more, the company serves mid-market and enterprise clients, offering comprehensive expertise in Cloud Migration, Data Platforms, DevOps, IoT, AI/ML, and more.
CloudThat is recognized as a top-tier partner with AWS and Microsoft, including the prestigious ‘Think Big’ partner award from AWS and the Microsoft Superstars FY 2023 award in Asia & India. Having trained 650k+ professionals in 500+ cloud certifications and completed 300+ consulting projects globally, CloudThat is an official AWS Advanced Consulting Partner, AWS Training Partner, AWS Migration Partner, AWS Data and Analytics Partner, AWS DevOps Competency Partner, Amazon QuickSight Service Delivery Partner, Amazon EKS Service Delivery Partner, Microsoft Gold Partner, AWS Microsoft Workload Partners, Amazon EC2 Service Delivery Partner, and many more.
To get started, go through our Consultancy page and Managed Services Package, CloudThat’s offerings.
FAQs
1. Can I catch multiple exceptions in one block?
ANS: – It is possible to handle several exceptions with a single block or to catch various errors with multiple unless blocks.
2. What is the else block's function in handling exceptions?
ANS: – If the try block finishes without raising any exceptions, the else block in exception handling runs the code.
3. When should I use finally block?
ANS: – The finally block is used for code, including cleanup operations, that needs to run whether an exception is raised.
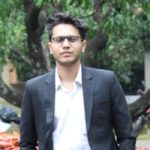
WRITTEN BY Raghavendra Santosh Kulkarni
Raghavendra is a skilled Full Stack Developer with expertise in a wide range of technologies. He has a strong working knowledge of AWS and is always looking to learn about new and emerging technologies. In addition to his technical skills, Raghavendra is a highly motivated and dedicated professional, committed to delivering high quality work.
Comments