Overview
As Steve Jobs mentioned once during his lifetime, “Everybody should learn how to code because programming teaches you how to use logic.”
That has inspired me since my early days of programming, but I wish I had heard it when I did not even know how to program. I would have considered programming a hobby instead of considering it a skill to enhance my grades and something required for a good career prospect. These days, coding has become a norm in schools and colleges, where it is frowned upon as an indispensable skill for students if they need to excel in IT because that is where the most high-paying jobs are. Whether that is the case or not is a matter of another discussion, but right now, I want to discuss things about programming that I wish I had known in my early days when I was beginning with programming. Most of these concepts relate to many languages that use Object Oriented Programming methodology, which is a more popular approach than procedural programming due to its simplicity in implementation and ability to relate to real-world scenarios. Some languages include C++, C# & Java, but since I am from a Microsoft background, I would focus on concepts directly applicable to C#.
C# is a popular language because of its use cases with the .NET framework by Microsoft, which is often used for web development and gamers to create XBOX and other PC-based games. You must know other tools like Unity Software and Unreal Engine to learn game development. You can even do some artwork designing for your games in case you want to go all the way, but that is not the agenda for this blog. Apart from other things, one thing that you essentially need to be strong in is programming concepts and mainly in Object Oriented Programming. So I will present insights from a recent lecture C# delivered internally by a fellow teammate to enhance our C# knowledge. Thanks to him, I learned some fantastic things about C# language and OOPS that I did not know even when working as a software developer. I would like to share my insights via this blog so that you learn these things early on, and it can help you in your programming career.
Handling Data Type Dilemma
One thing many programmers get mixed up, which I am also used to, is when to use short, Int, and long. So, it is very important to understand the difference between these variable types and when to use them, as it will help you avoid errors when initializing variables but will also help you to reduce costs in the long run. Short, Int, and long have ranges in which they can be assigned to values of integers. For example, if you want to assign a value of a lower number range, ‘short’ is the best choice. Numbers higher than that can be assigned as Int, and even higher numbers can be assigned as long. Now, this is important because if something requires a shorter memory space. You assign it as Int or long; the rest of the memory is getting under-utilized and adding to the cost of your servers. On the other hand, if you need to assign short or Int to a large number that was supposed to be long, it will fall out of memory and give you a compile error as it can only take specific bytes of data.
- Cloud Migration
- Devops
- AIML & IoT
Explicit and Implicit Conversion Confusions
The concept of implicit and explicit conversion was the next thing when it came to variables that I was not even aware existed for a long time. Implicit conversion is when one data type is to be converted to another data type, and the conversion is compatible, so it does not need any type casting. Explicit conversion is done when the data types are incompatible, and you need to convert the data to another type. In that case, you explicitly convert one data type to another. The important thing to note here is that people often do not understand the difference and do explicit conversion even when implicit conversion is possible. You should avoid that trap and always check before going for explicit conversions if data types are compatible.
Minimizing Chaos with Operators
Enough about data types and variables. Let’s talk about operators. Specifically && (And) operator, which we think we know how to use but we necessarily might not. The && operator returns true when both operands return true, just like the
|| (Or)
operator, but when any of the expressions are false, it is supposed to return false. Now, it does that, but you did not know that it does not check for the right expression if the left expression is false and directly returns false. This method makes sense since the result will be false no matter what is on the right side. If you don’t believe me, do test it out for yourself.
Working with Console.Readline()
A function we often use to get user input is the Console.Readline()
. Did you know that by default, it accepts a string as the parameter, and whatever you enter as text, even if it is a number, would be converted to a string format to send to the run time compiler. That is why if you need to use the input entered by the user to the console further in the program, you need to convert it to int32, and then you would be able to use it for performing further operations.
Using Short Cuts to Write Comments
Many people struggle with commenting and uncommenting a bunch of code, and they usually use
'/* */'
for multi-line comments. I used to do that as well, but there is a better way. Using shortcut keys Ctrl+K+C & Ctrl+K+U
one can easily comment or uncomment multiple lines of code after highlighting it.
Working with Console.Writeline( )
One difference that people often struggle with is where to use the console.writeline()
and console.write()
. The only difference between both is that console.writeline()
will print the output in the next line and console.write()
will output the result in the same line. I know it’s not much, but it can sometimes get confusing.
Handling switch() Statement Smartly
One interesting functionality in OOPS-based languages is the switch()
statement, which is not as used in a production scenario. One thing few people observe in switch()
cases is that if you use break in a case, it will immediately terminate the entire switch()
block, but if you do not use the break keyword, you will get an error. This we already knew, but what we did not know is that if you add a blank case, i.e., just write the name of the case with a : (for ex: case0: ) and then add the next case, it simply will jump to the next case and keep on executing all the consequent cases unless it finds a non-empty case or the switch()
block ends. Fascinating, isn’t it?
Utilizing the 'new' Keyword Effectively
Nobody told me the use of the ‘new
‘ Keyword when creating an object that must be used only for instantiating new objects. Have you tried instantiating new objects without using the new Keyword. The funny part is that it will work, only that when you use the new Keyword, it creates a new object in the memory and allocates space to all the variables in that class, which are created along with the object. So if you want to continue using the same object without instantiating a new object and allocating fresh space for the variables of the new object, instantiate the object without using the new Keyword. Be careful; you are working with the same object, which can be overridden.
Handling 'Null Value' Properly
If you do not assign any value to a variable, it gets assigned a default value of 0. The same is true with variables that are created by instantiating an object. However, if you assign a null value to the entire object and then try to use the object with a function, it will throw an error immediately.
Using 'Properties' Diligently
One of my personal nemesis in C# was the use of properties and how we use them or why we require them in the first place. Now I understand that properties just assign value to the variables without passing it through a constructor or when you want to directly assign the value later dynamically rather than a static initialization. Also, encapsulation is a very important concept where properties play a major role. With encapsulation, you can make the set method inside a property as ‘private’ and then assign the value to the variable you are setting in the property like ‘private set num = 2
‘. This step will ensure that people can get the value for the property but cannot set it without permission directly from the class.
Working with the 'static' Keyword
We discussed earlier how the new Keyword will create a new memory allocation for an object. We can ensure only a single memory allocation is created by using the ‘static
‘ Keyword. Static will ensure that the object that gets instantiated cannot be assigned to another object, but still, the value for the variables inside the static object can be changed.
Best practice of handling Exceptions-1
A best practice everyone tells us about is using the try-catch
blocks to handle exceptions, but many people use them incorrectly. Whenever a try block is used, and an error is thrown by it, the catch block handling the exception should also be of the correct type. Using a catch block with a different type of exception-handling function will still throw an error. Most people do not do this; instead, they prefer to use the general message exception function in the catch block. This method is not recommended when you want your users to get the correct information about the kind of exception they encountered.
Best practice of handling Exceptions-2
There is also a chance that the catch block you are using to handle the exception might generate an exception. To prevent it, you should even handle any exceptions that may arise inside the catch block, which can be done using nested try-catch
blocks.
File Handling Best Practices
File handling is an advanced topic that people usually use in specific scenarios, so everyone might not be aware of its best practices. One such best practice is when you use the FileStream
& StreamWriterReader
class, you activate a lock to the file being opened or read/written to. If others try to access that file when the lock is activated, they will get an error. So, once you are done modifying or reading the file, you should use the .close()
method along with the FileStream & StreamReader/Writer objects to deactivate the lock.
Working with the 'delegates' concept
The last concept that flies over the top of the head is delegates. If you are familiar with delegates
, you might still struggle with using them, but you need to know that if you add the same method again to the delegate, it will treat it as a separate object and re-compute it.
Conclusion
These were some best practices and fresh insights regarding C# as a language. It comes down to how you are using them if you are eager to upskill your coding skills and grow as a Full Stack Developer.
CloudThat offers comprehensive training on Full Stack Development, Programming, Cloud-related certification training, and DevOps sessions for individuals, students, and corporates. Get in touch with us to learn more about it.
Get your new hires billable within 1-60 days. Experience our Capability Development Framework today.
- Cloud Training
- Customized Training
- Experiential Learning
About CloudThat
CloudThat has led the deck in India’s Cloud Consulting & Cloud Training Arena since 2012. This year we achieved a remarkable feat as the Microsoft Asia & India Super Star Campaign winner -FY’23.
We are uniquely recognized as the Microsoft Solutions Partner, AWS Authorized Training Partner, AWS Partner Advanced Tier Services, and Authorized VMware Training Reseller.
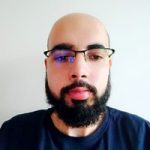
WRITTEN BY Rishabh Beniwal
Comments